系统栈与递归之间的联系 ,以及用栈来模拟系统栈写出非递归程序
系统栈与递归之间的关系
图解系统栈与递归之间的关系:
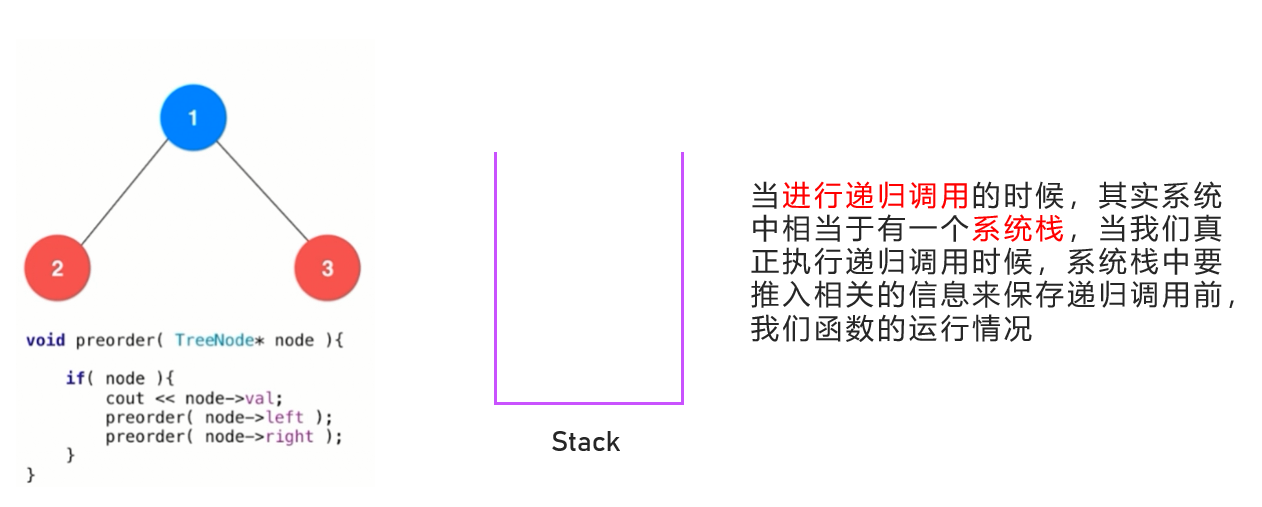
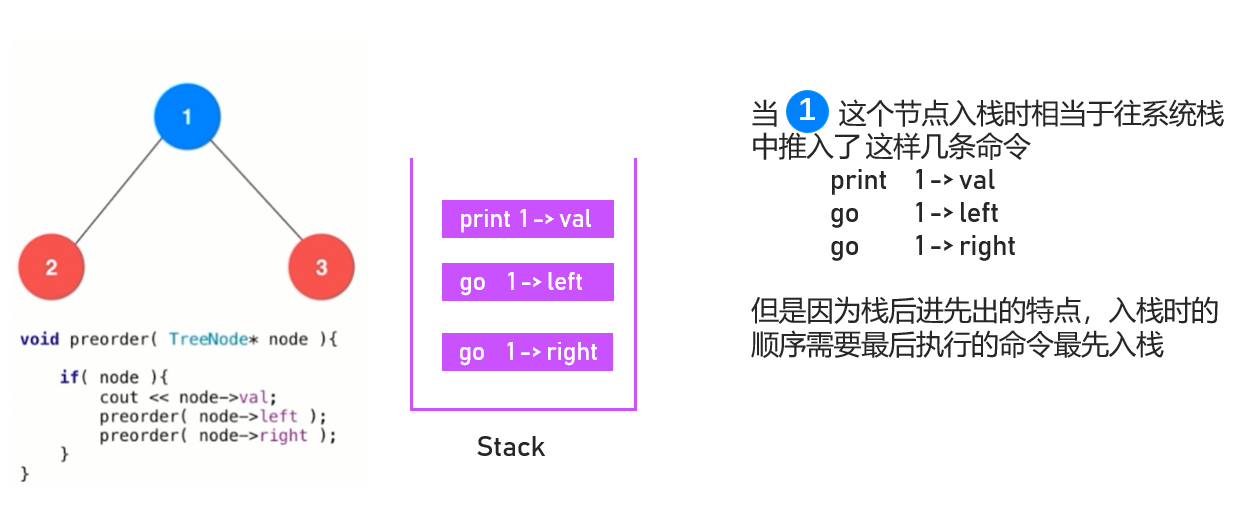
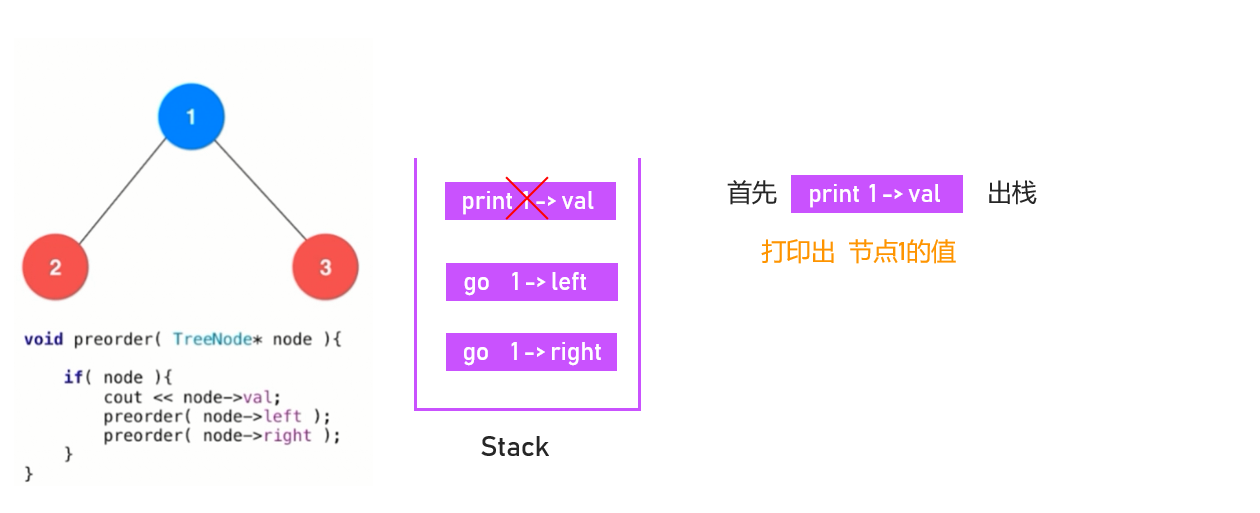
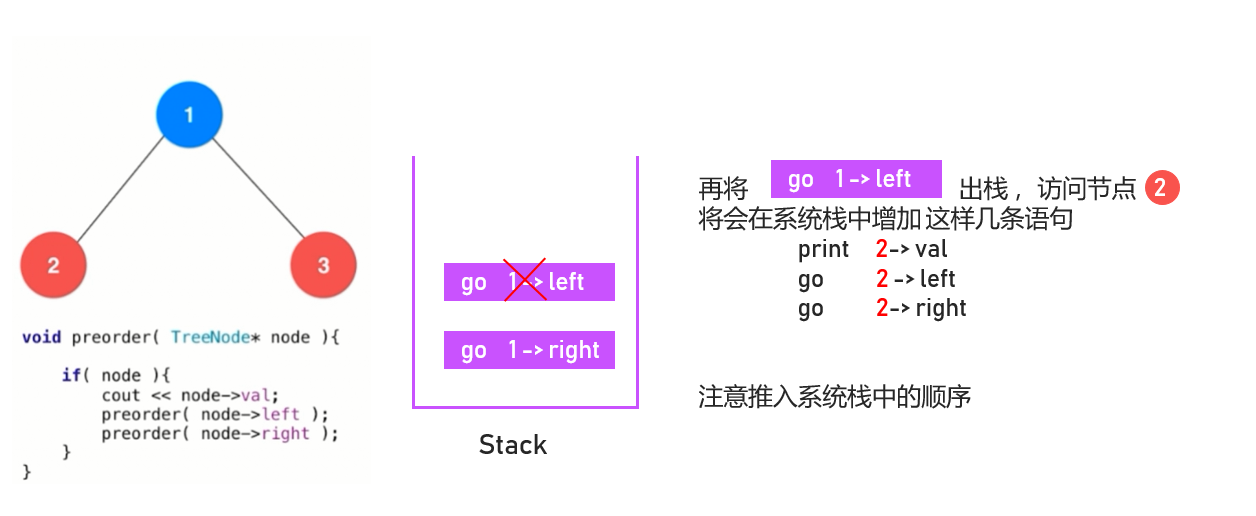
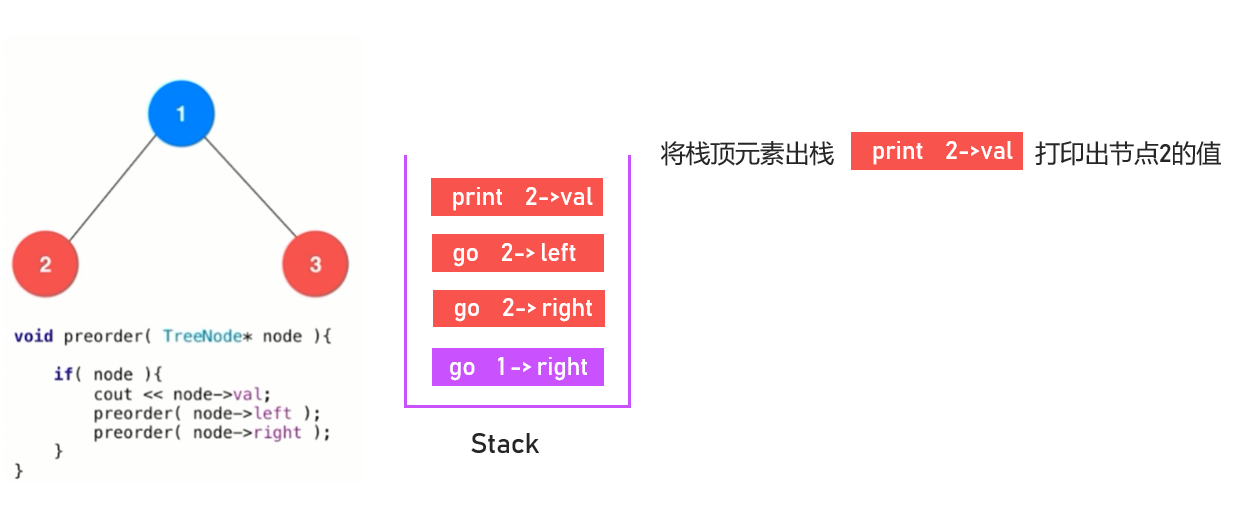
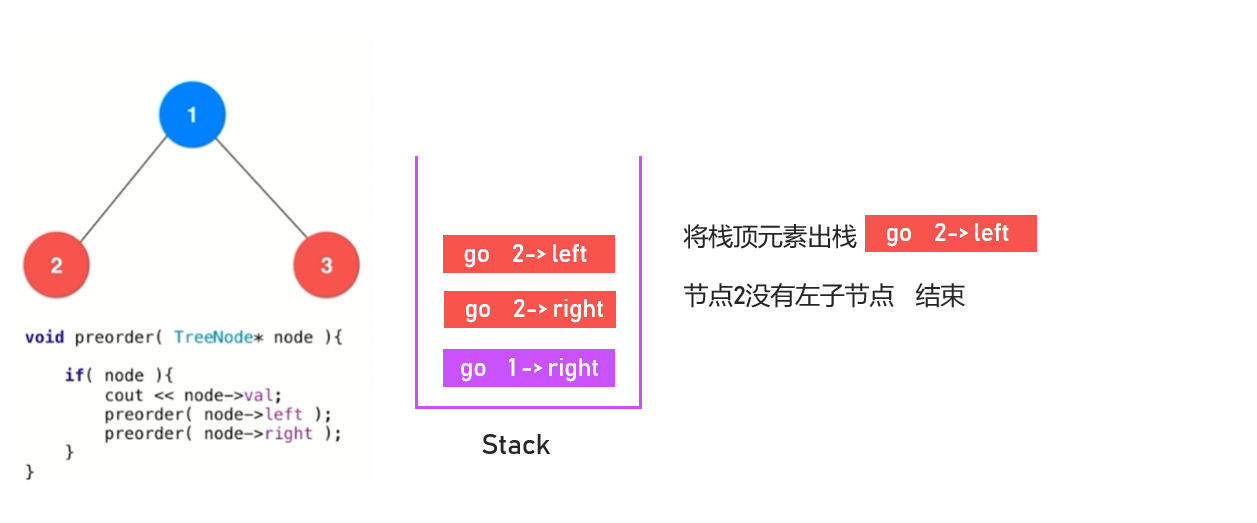
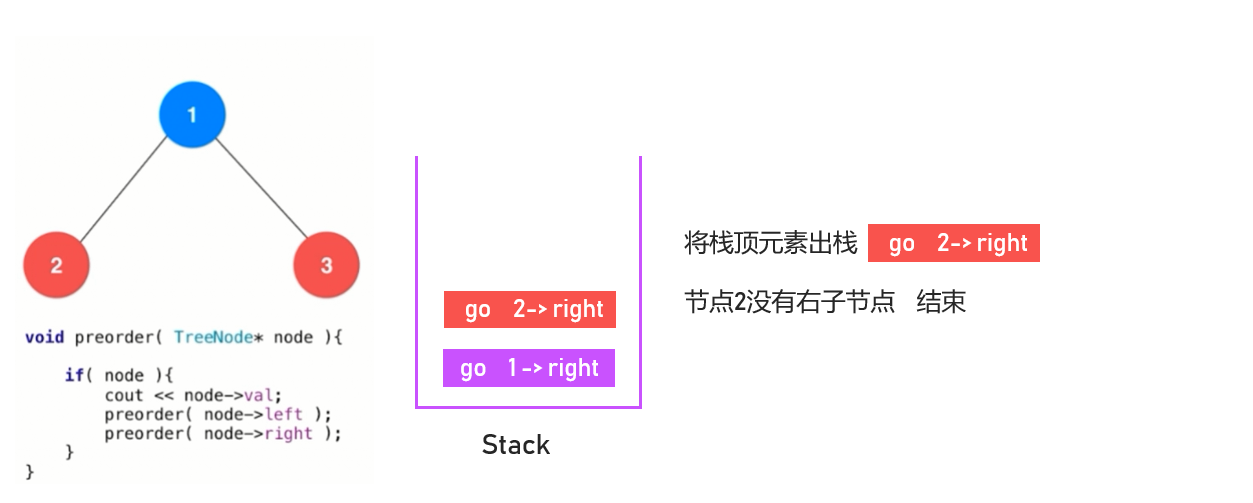
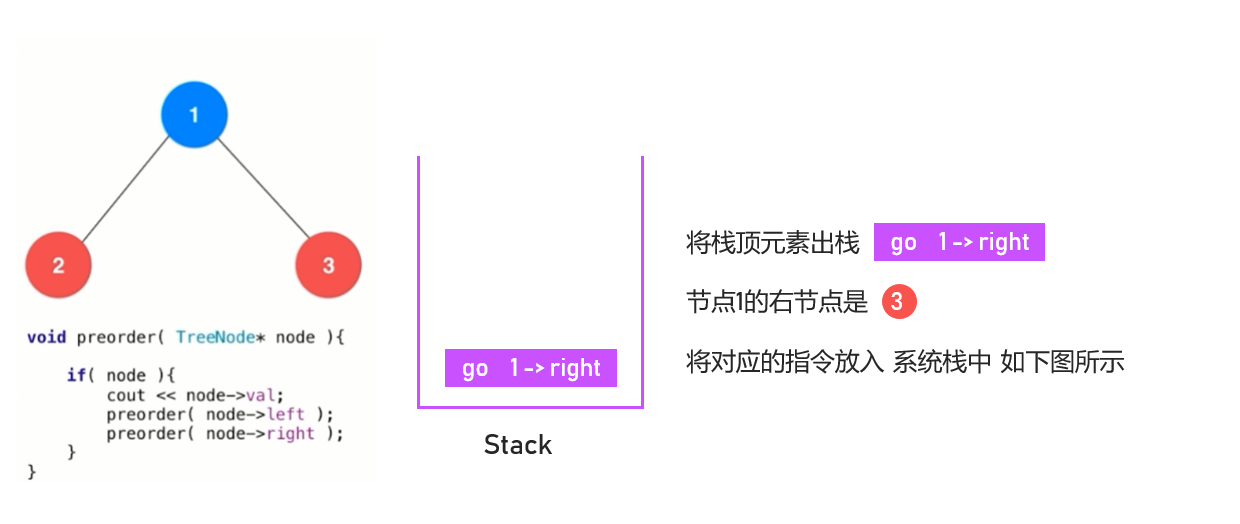
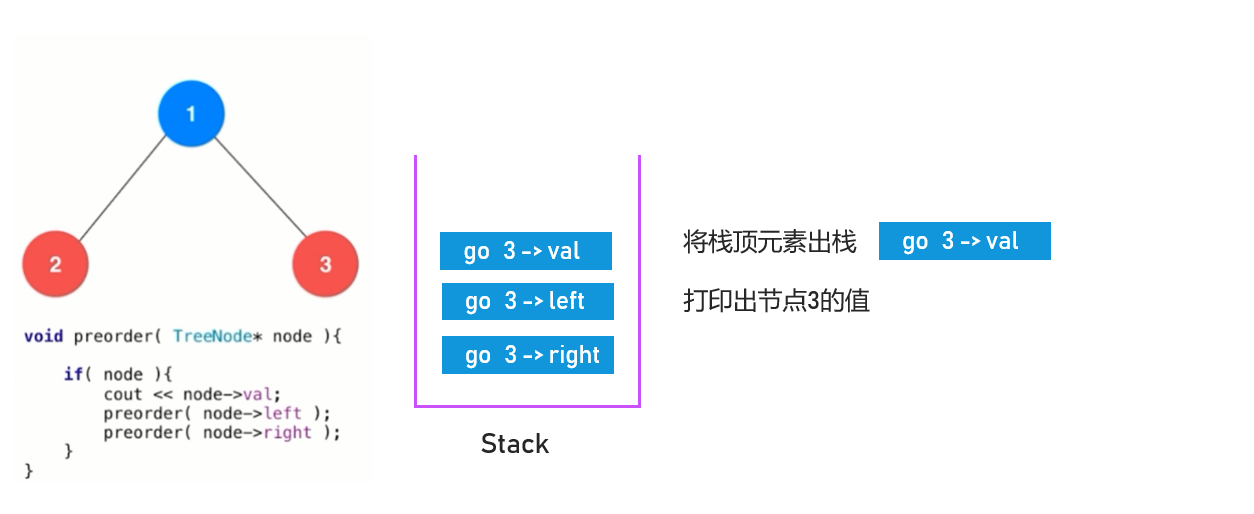
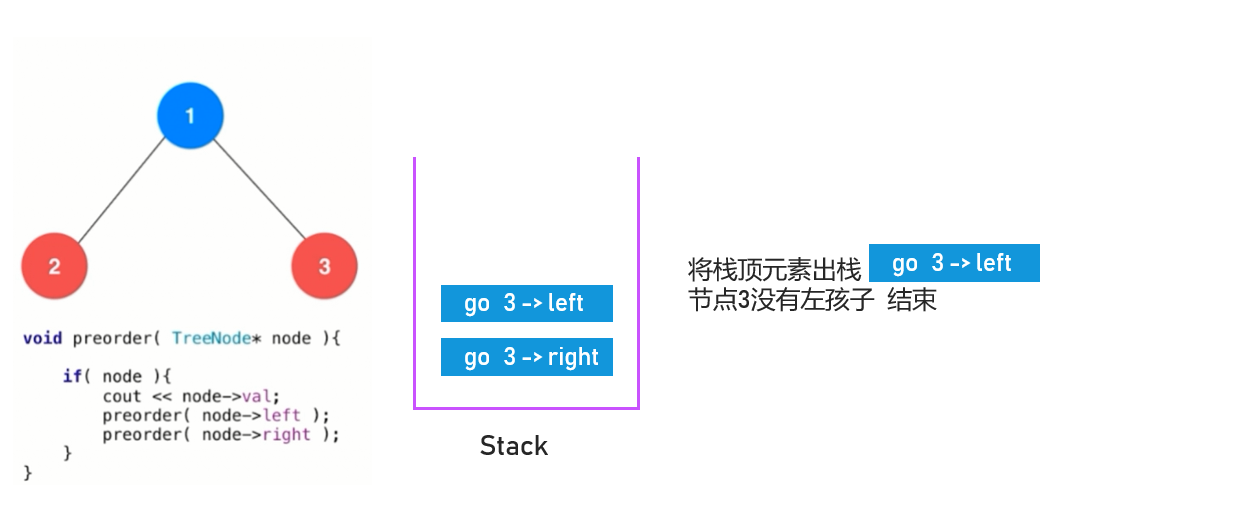
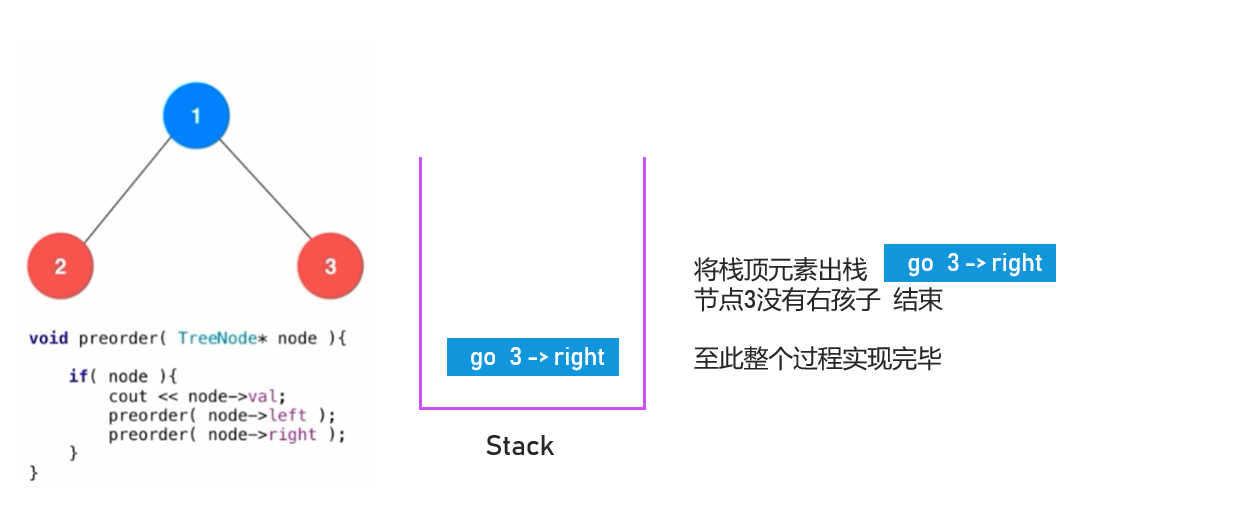
用栈来模拟系统栈 写出非递归程序
举个栗子 leetcode 144 Binary Tree Preorder Traversal:题目链接递归遍历写法 AC:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public List<Integer> preorderTraversal(TreeNode root) {
ArrayList<Integer> array = new ArrayList<Integer>();
preorderTraversal( root , array);
return array;
}
public void preorderTraversal(TreeNode root, List<Integer> array) {
if (root != null) {
array.add(root.val);
preorderTraversal(root.left, array);
preorderTraversal(root.right, array);
}
}
}
用栈来模拟系统栈 非递归 AC (推荐):
1 | /** |
leetcode 94. Binary Tree Inorder Traversal:题目链接
leetcode 145. Binary Tree Postorder Traversal:题目链接