- Minimum Depth of Binary Tree:题目链接
BFS
思路:
借助队列首先根节点入队,此时depth=1,然后将depth=1的结点出队,出队的同时将depth=1的结点的左右孩子入队,此时depth = 2,将depth = 2的结点出队并将其左右孩子入队,同理第N层也是这样,在此过程中检查是否有结点是叶节点,有说明该叶节点对应的深度就是最小深度1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29class Solution {
// BFS 时间复杂度O(N) 空间复杂度O(M) M:队列的长度
public int minDepth(TreeNode root) {
if (root == null) { // 树空
return 0;
}
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root); // 根节点入队
int depth = 0; // 二叉树最小深度 初始为0
while (!queue.isEmpty()) { // 队列不空
depth++; // 深度+1
// 获取当前层次的所有结点 并将其左右孩子入队
for (int size = queue.size(); size > 0; --size) {
TreeNode front = queue.poll(); // 取出队首元素
if (front.left == null && front.right == null) { // 如果队首元素是叶节点说明 该结点所在层次即为最小深度
return depth;
}
if (front.left != null) { // 左孩子不空 左孩子入队
queue.offer(front.left);
}
if (front.right != null) { // 右孩子不空右孩子入队
queue.offer(front.right);
}
}
}
return depth;
}
}
解法2:
BFS入队列的是一个新建的结构体里面包含当前结点已经当前结点所在深度1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42class Solution {
private class Node{
TreeNode node;
int level;
public Node(){
this(null,0);
}
public Node(TreeNode node,int level ){
this.node = node;
this.level = level;
}
}
public int levelOrder(TreeNode root) {
int res = 999999;
if (root == null) {
return 0;
}
Queue<Node> queue = new LinkedList<>();
queue.offer(new Node(root,1));
while (!queue.isEmpty()) {
Node front = queue.poll();
if (front.node.left==null && front.node.right==null && front.level < res) {
return front.level;
}
if (front.node.left != null) {
queue.add(new Node(front.node.left, front.level + 1));
}
if (front.node.right != null) {
queue.add(new Node(front.node.right, front.level + 1));
}
}
return res;
}
public int minDepth(TreeNode root) {
return levelOrder(root);
}
}
DFS-递归
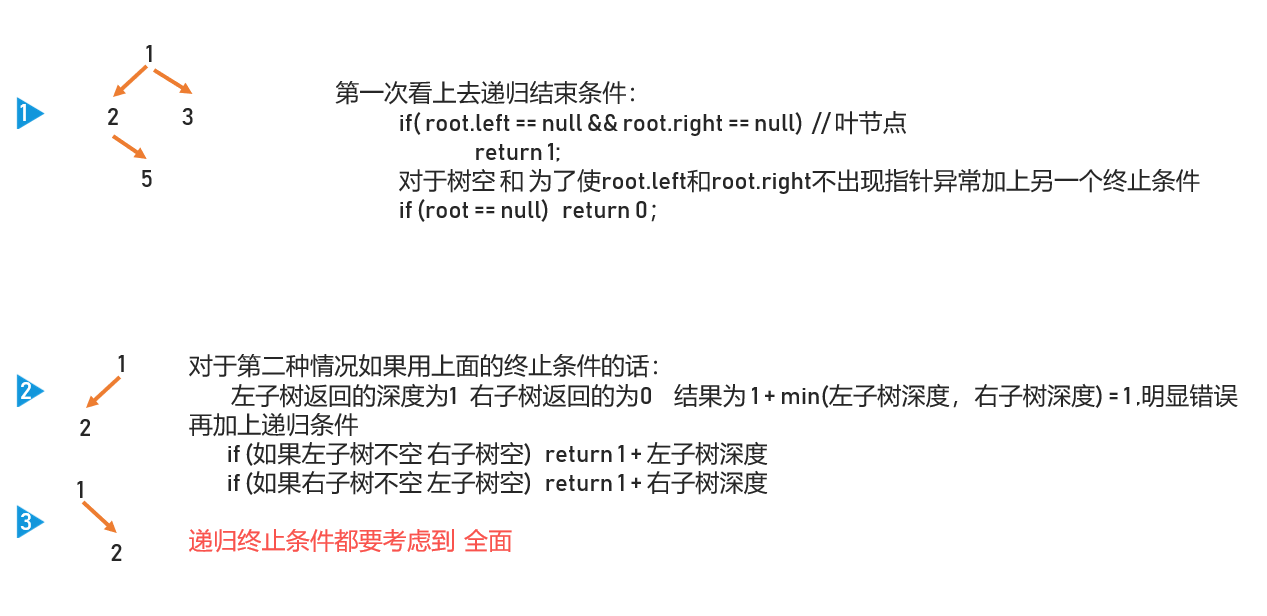
1 | class Solution { |